Offensive Development with C++ and C#: Process Injection
RedTeam Malware Development Offensive Development Win32 API Remote Thread Injection Process Hollowing DLL Injection Process Injection
Introduction to Process Injection in Windows
In my previous post, we explored the fundamentals of the Win32 API and in our first code in C++, laying a foundation for understanding how applications interact with the Windows operating system. Today, we will delve into a more advanced and exciting topic: process injection. This technique is commonly used in malware development to execute code within the address space of another process, allowing for stealthy operations and evasion of security mechanisms.
Learning Objectives
By the end of this article, you will be able to:
- Understand the concept and purpose of process injection.
- Identify and describe various process injection techniques.
- Recognize the use cases and implications of process injection in offensive development
Before Starting, we are going to recap the previous blog post
Recap: Getting Started with Win32 API and C++ Development
Before we dive into process injection, let’s quickly recap what we covered in the first article:
- Introduction to the Win32 API
- Key DLLs and their functionalities
- User Mode vs. Kernel Mode in Windows OS
- Why we use C++ ou C#
- Practical examples including the
MessageBox
function and process Enumeration example in C++.
If you missed the first post, you can check it out here.
Understanding Process Injection
In offensive development, one of the most effective technique employed is process injection. This method has become increasingly prevalent, especially in advanced persistent threats (APTs) and sophisticated malware campaigns. Process injection involves inserting code into a running process. This can be done using various techniques, each with its own advantages and complexity. The main goal is to run arbitrary code within the context of another process like notepad.exe
or explorer.exe
, which can be useful for tasks like evading security measures or performing covert operations. This technique usually consists of a chain of three primitives.
- Allocation primitive : Used to allocate memory
- Writing primitive: Used to write malicious code to the allocated memory
- Execution primitive: Used to execute the malicious code written
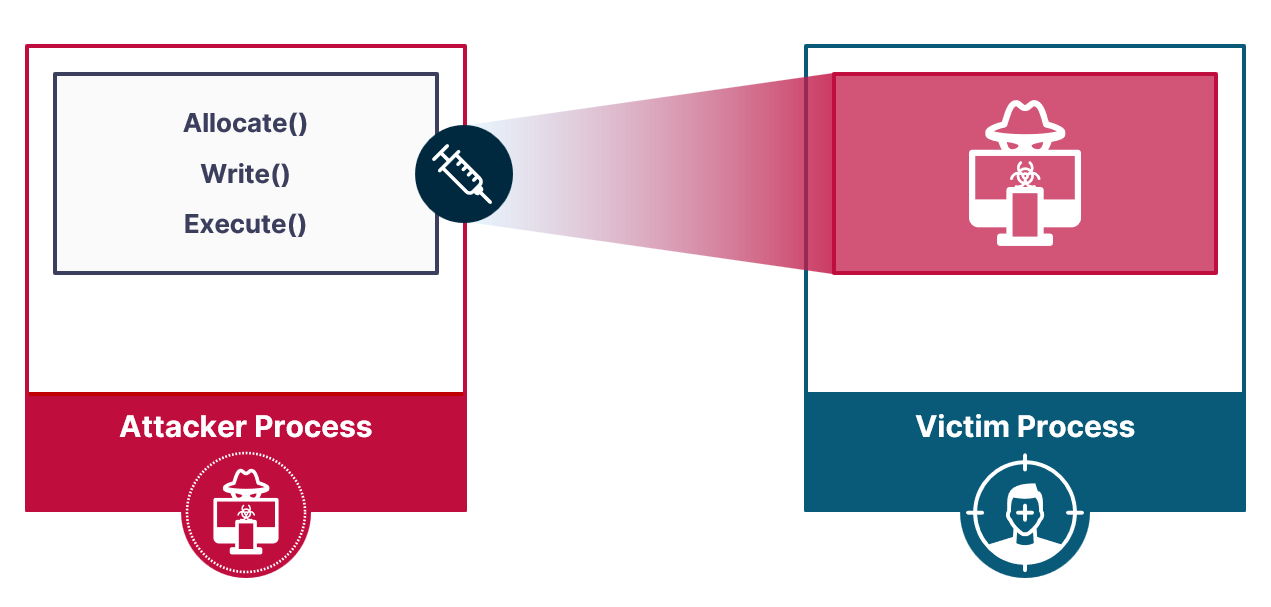
NB: The most basic injection technique would use the Win32 API functions VirtualAllocEX() for allocation, WriteProcessMemory() for writing, and CreateRemoteThread() for execution.
Common Process Injection Techniques
Let’s talk about some process injection techniques
1. Remote Thread Injection
This is one of the most straightforward methods. It simply involves creating a remote thread in the target process to execute the injected code.
Steps:
- Open the target process by name like
notepad.exe
usingOpenProcess
. - Allocate memory in the target process using
VirtualAllocEx
. - Write the payload (code) into the allocated memory using
WriteProcessMemory
. - Create a remote thread in the target process with
CreateRemoteThread
.
Advantages:
- Simple to Implement
- Widely supported across different versions of windows
Diagram:
Process A Process B
(malware.exe) (notepad.exe)
| |
| OpenProcess() |
|------------------->|
| |
| VirtualAllocEx() |
|------------------->|
| |
| WriteProcessMemory()|
|------------------->|
| |
| CreateRemoteThread()|
|------------------->|
| |
v v
Code executed in Process B
2. DLL Injection
This technique involves injecting or loading a DLL(Library or .dll file) into the target process and executing its code.
Steps:
- Open the target process.
- Allocate memory for the DLL path in the target process.
- Write the DLL path into the allocated memory.
- Use
CreateRemoteThread
to callLoadLibrary
with the DLL path as an argument.
Advantages:
- DLLs can be easily reused and shared.
- Allows complex functionality to be injected.
- Can easily AVs as the target process is a legitimate one
Diagram:
Process A Process B
(malware.exe) (notepad.exe)
| |
| OpenProcess() |
|--------------------->|
| |
| VirtualAllocEx() |
|--------------------->|
| |
| WriteProcessMemory() |
|--------------------->|
| |
| CreateRemoteThread() |
|--------------------->|
| |
v v
DLL loaded and executed in Process B
3. APC (Asynchronous Procedure Call) Injection
We Inject a shellcode in to a remote thread then queue an Asynchronous Procedure Call
Object to this thread within . When the thread enters an alertable state(when it calls SleepEx
, SignalObjectAndWait
, MsgWaitForMultipleObjectsEx
, WaitForMultipleObjectsEx
), the Asynchronous Call
is executed.
This technique is similar to the Thread Hijacking technique
Steps:
- Open the target process and target thread.
- Allocate memory for the payload in the target process.
- Write the payload into the allocated memory.
- Queue the APC to the target thread using
QueueUserAPC
.
Advantages:
- Can be used to execute code in specific threads.
- Often bypasses basic security mechanisms as it reuses existing threads
Diagram:
Process A Process B
(malware.exe) (notepad.exe)
| |
| OpenProcess() |
|----------------------->|
| |
| OpenThread() |
|----------------------->|
| |
| VirtualAllocEx() |
|----------------------->|
| |
| WriteProcessMemory() |
|----------------------->|
| |
| QueueUserAPC() |
|----------------------->|
| |
| CreateRemoteThread() |
|----------------------->|
v v
APC executed in Process B's thread
4. Process Hollowing
In process hollowing, a new process is created in a suspended state, its memory is unmapped, and the memory of the target executable is mapped into it. Then we resume the process in suspended state.
Steps:
- Create a new process (
svchost.exe
) in a suspended state usingCreateProcess
. - Read the .text bloc to look for image base pointer of thre created process.
- Allocate memory for the target executable in the new process.
- Write the target executable into the allocated memory.
- Resume the process.
Advantages:
- Harder to detect as the original process name remains unchanged.
- Effective for executing large payloads.
Diagram:
Process A Process B
(malware.exe) (svchost.exe)
| |
| CreateProcess() (suspended)|
|-------------------------->|
| |
| ReadProcessMemory() |
|-------------------------->|
| |
| VirtualAllocEx() |
|-------------------------->|
| |
| WriteProcessMemory() |
|-------------------------->|
| |
| ResumeThread() |
|-------------------------->|
| |
v v
Target executable runs in Process B
5. Thread Hijacking
Thread hijacking involves suspending a thread in the target process, modifying its context to point to the injected code, and then resuming the thread. It’s similar to process Hollowing but concerns threads only.
Steps:
- Open the target process and suspend a thread using
SuspendThread
. - Allocate memory for the payload in the target process.
- Write the payload into the allocated memory.
- Modify the thread context to point to the injected code using
SetThreadContext
. - Resume the thread using
ResumeThread
.
Advantages:
- Utilizes existing threads, reducing detection risk.
- Efficient for quick code execution.
Diagram:
Process A Process B
(malware.exe) (notepad.exe)
| |
| OpenProcess() |
|--------------------------->|
| |
| SuspendThread() |
|--------------------------->|
| |
| VirtualAllocEx() |
|--------------------------->|
| |
| WriteProcessMemory() |
|--------------------------->|
| |
| SetThreadContext() |
|--------------------------->|
| |
| ResumeThread() |
|--------------------------->|
| |
v v
Hijacked thread runs injected code in Process B
6. Inter-Process Mapping Section
This technique involves creating a new memory section and mapping a view of this section locally. The shellcode is copied into the local mapped view, and a remote mapped view of this local view is then created in the target process. So the first map is only used for alteration and the second map for execution to avoid suspucious memory space. Finally, a new thread is created in the target process with the mapped view as its entry point..
Steps:
- Create a section object using
CreateFileMapping
. - Map the section into the address space of the current process using in Read and Write protection
MapViewOfFile
. - Write the payload into the allocated memory.
- Open the target process.
- Map the section into the address space of the target process using in Read and Execute protection
MapViewOfFile2
. - Create a remote thread in the target process with
CreateRemoteThread
.
Advantages:
- Efficient for large data transfers.
- Provides a persistent communication channel between processes.
- Helps to avoid AV as the first mapped memmory are only in Read & Write (RW) protection and the second is on Read & Execute(RX) protection. (No Read, Write & Execute Simultaneously -> No RWX)
Diagram:
Process A Process B
| |
| CreateFileMapping() |
|---------------------------->|
| |
| MapViewOfFile(RW) |
|---------------------------->|
| |
| VirtualAllocEx() |
|---------------------------->|
| |
| WriteProcessMemory() |
|---------------------------> |
| |
| OpenProcess() |
|---------------------------->|
| |
| MapViewOfFile2(RX) |
|---------------------------->|
| |
| CreateRemoteThread() |
|---------------------------->|
| |
v v
Shared memory accessible in both processes
7. Other Injection Techniques
There are other injection techniques more sophisticated like Reflective DLL Injection, Atom Bombing, Process Doppel Ganging, etc. But one blog article can’t be enough to present all theses techniques.
Conclusion
Process injection is a powerful technique in offensive security, offering various methods to execute code within the context of another process. Understanding these techniques is crucial for developing advanced security tools and understanding potential attack vectors.
Future posts will continue to explore these methods in more detail, providing practical examples and further insights into their use cases and detection. Specifically, the next post will focus on some pratical process injection examples in C# and C++.
Stay tuned for more in-depth tutorials and examples. If you have any questions or topics you would like me to cover, feel free to reach out!
References
#Infosec #CyberSecurity #ProcessInjection #Win32API #C++ #MalwareDevelopment #RedTeam #CyberCommunity #RemoteProcessInjection